Bitmap
Pixel Collision
By:
Rohan Varma
Introduction:
This tutorial will walk you
through creating a method that will be able to detect when two images collide,
not just their Rects. The tutorial assumes that you are able to load and draw
two bitmaps onto the screen and that you are familiar with the Rect.intersect()
method. If you are not, refer to the tutorials that teach you these things
before continuing further.
Create the project
Create a new project and set
it up so that there are two bitmaps moving around the screen
Detecting collision
- Detecting when two bitmaps collide
requires two steps. The first is to find the overlapping area between both
bitmaps’ Rects. Once the overlapping rectangle has been found, we iterate
through each pixel in it and check to see if both images are not
transparent at that pixel (any color can be used, but I use transparent
because my pictures have a transparent background and if one of the two
images is transparent at a given pixel, it means the bitmaps are not
colliding at that pixel)
- Create a Boolean method with
parameters
.Have
it return false at the bottom


- Finding the overlapping rectangle is
really easy, as Android calculates it for you through the Rect.intersect()
method. The thing is, the Rect.intersect() method is a destructive call,
meaning that it changes one of the Rects you put into it. What I mean by
this is if you call
,
Android will actually change r1 to the overlapping rectangle. Since we will
need to know what r1 originally is, the first step is accomplished by creating
a temporary rectangle and setting it equal to r1 and then using that to call
the Rect.intersect() method. In order to do this your method should now look
like this.
- Now comes the second step. We have
found the overlapping rectangle and now we need to iterate through its pixels,
checking to see if both images are not transparent at any of the pixels.
We implement this iteration by putting the follo
wing
for loops inside the if statement of the method
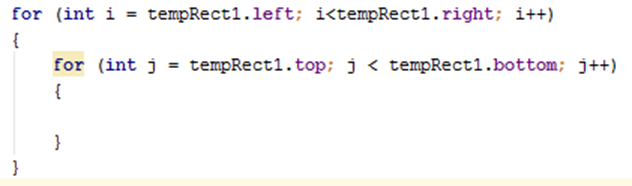
- To check whether or not the images
are transparent at a given pixel, we use the Bitmap.getPixel(x, y) method.
However, an important thing to note about the Bitmap.getPixel(x, y) method
is that the coordinate point (0, 0) starts at the top left corner of the
bitmap, not the top left corner of the screen. On the other hand, the x
and y (i and j from the for loop) values you get if you use the
overlapping rectangle found earlier start with (0, 0) in the top left
corner of the screen. Therefore, to avoid throwing an
IllegalArgumentException, we subtract the top of the bitmap from i and the
left of the bitmap from j. We check the color of the pixel in both bitmaps
and if both are not transparent then a collision has happened and we
return true. To do all this, we add the following if statement inside the
nested for loops.

- Your method is now complete!! It
should now look like this
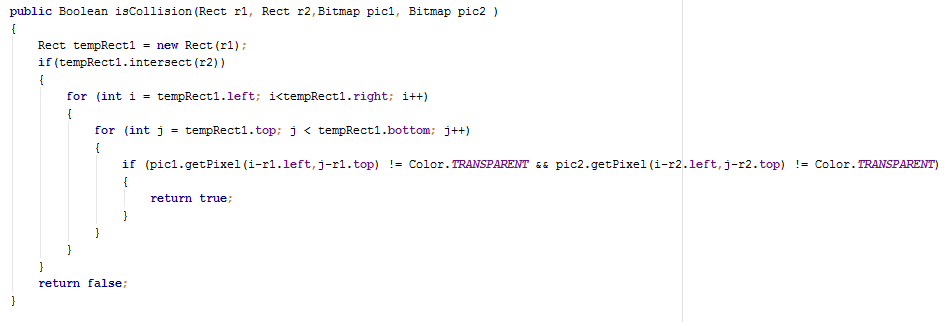
You
can add this into any program, pass in the appropriate parameters, and see if
two bitmaps collide, not just their Rects!